Java 7: A Comprehensive Tutorial
by Budi Kurniawan
ISBN: 9780980839661
$59.99, 846 pages
by Budi Kurniawan
ISBN: 9780980839661
$59.99, 846 pages
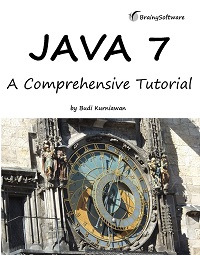
This book covers the most important topics any Java developer should master: object-oriented programming, Java language syntax, and the Java libraries. Designed as a guidebook for those who want to become a Java developer, Java 7: A Comprehensive Tutorial discusses the essential Java programming topics that you need to master in order teach other technologies to yourself.
Table of Contents
Introduction - Java, the Language and the Technology - An Overview of Object-Oriented Programming - About This Book - Downloading and Installing Java - Downloading Program Examples and Answers Chapter 1: Your First Taste of Java - Your First Java Program - Java Code Conventions - Integrated Development Environments (IDEs) - Summary - Questions Chapter 2: Language Fundamentals - ASCII and Unicode - Separators - Primitives - Variables - Constants - Literals - Primitive Conversions - Operators - Comments - Summary - Questions Chapter 3: Statements - An Overview of Java Statements - The if Statement - The while Statement - The do-while Statement - The for Statement - The break Statement - The continue Statement - The switch Statement - Summary - Questions Chapter 4: Objects and Classes - What Is a Java Object? - Java Classes - Creating Objects - The null Keyword - Objects in Memory - Java Packages - Encapsulation and Access Control - The this Keyword - Using Other Classes - Final Variables - Static Members - Static Final Variables - Static import - Variable Scope - Method Overloading - By Value or By Reference? - Loading, Linking, and Initialization - Object Creation Initialization - Comparing Objects - The Garbage Collector - Summary - Questions Chapter 5: Core Classes - java.lang.Object - java.lang.String - java.lang.StringBuffer and java.lang.StringBuilder - Primitive Wrappers - Arrays - java.lang.Class - java.lang.System - java.util.Scanner - Boxing and Unboxing - Varargs - The format and printf Methods - Summary - Questions Chapter 6: Inheritance - An Overview of Inheritance - Accessibility - Method Overriding - Calling the Superclass's Constructors - Calling the Superclass's Hidden Members - Type Casting - Final Classes - The instanceof Keyword - Summary - Questions Chapter 7: Error Handling - Catching Exceptions - try without catch - Catching Multiple Exceptions - The try-with-resources Statement - The java.lang.Exception Class - Throwing an Exception from a Method - User-Defined Exceptions - Final Words on Exception Handling - Summary - Question Chapter 8: Numbers and Dates - Number Parsing - Number Formatting - Number Parsing with java.text.NumberFormat - The java.lang.Math Class - The java.util.Date Class - The java.util.Calendar Class - Date Parsing and Formatting with DateFormat - Summary - Questions Chapter 9: Interfaces and Abstract Classes - The Concept of Interface - The Interface, Technically Speaking - Base Classes - Abstract Classes - Summary - Questions Chapter 10: Enums - An Overview of Enum - Enums in a Class - The java.lang.Enum Class - Iterating Enumerated Values - Switching on Enum - Summary - Questions Chapter 11: The Collections Framework - An Overview of the Collections Framework - The Collection Interface - List and ArrayList - Iterating Over a Collection with Iterator and for - Set and HashSet - Queue and LinkedList - Collection Conversion - Map and HashMap - Making Objects Comparable and Sortable - Summary - Questions Chapter 12: Generics - Life without Generics - Introducing Generic Types - Using Generic Types without Type Parameters - Using the? Wildcard - Using Bounded Wildcards in Methods - Writing Generic Types - Summary - Questions Chapter 13: Input/Output - File Systems and Paths - File and Directory Handling and Manipulation - Input/Output Streams - Reading Binary Data - Writing Binary Data - Writing Text (Characters) - Reading Text (Characters) - Logging with PrintStream - Random Access Files - Object Serialization - Summary - Questions Chapter 14: Nested and Inner Classes - An Overview of Nested Classes - Static Nested Classes - Member Inner Classes - Local Inner Classes - Anonymous Inner Classes - Behind Nested and Inner Classes - Summary - Questions Chapter 15: Swing Basics - AWT Components - Useful AWT Classes - Basic Swing Components - Summary - Questions - Chapter 16: Swinging Higher - Layout Managers - Event Handling - Working with Menus - The Look and Feel - Fast Splash Screens - System Tray Support - Desktop Help Applications - Summary - Questions Chapter 17: Polymorphism - Defining Polymorphism - Polymorphism in Action - Polymorphism in a Drawing Application - Polymorphism and Reflection - Summary - Questions Chapter 18: Annotations - An Overview of Annotations - Standard Annotations - Common Annotations - Standard Meta-Annotations - Custom Annotation Types - Summary - Questions Chapter 19: Internationalization - Locales - Internationalizing Applications - An Internationalized Swing Application - Summary - Questions Chapter 20: Applets - A Brief History of Applets - The Applet API - Security Restrictions - Writing and Deploying Applets - How AppletViewer Works - Passing Parameters to an Applet - SoundPlayerApplet - JApplet - Applet Deployment in a JAR File - Faster Loading - Summary - Questions Chapter 21: Java Networking - An Overview of Networking - The Hypertext Transfer Protocol (HTTP) - java.net.URL - java.net.URLConnection - java.net.Socket - java.net.ServerSocket - A Web Server Application - Summary - Questions Chapter 22 : Java Database Connectivity - Introduction to JDBC - Four Steps to Data Access - Closing JDBC Objects - Reading Metadata - The SQLTool Example - Summary - Questions Chapter 23: Java Threads - Introduction to Java Threads - Creating a Thread - Working with Multiple Threads - Thread Priority - Stopping a Thread - Synchronization - Visibility - Thread Coordination - Using Timers - Swing Timers - Summary - Questions Chapter 24: Concurrency Utilities - Atomic Variables - Executor and ExecutorService - Callable and Future - Swing Worker - Locks - Summary - Questions Chapter 25: Security - Java Security Overview - Using the Security Manager - Policy Files - Permissions - Using the Policy Tool - Applet Security - Programming with Security - Cryptography Overview - Creating Certificates - The KeyTool Program - The JarSigner Tool - Java Cryptography API - Summary - Questions Chapter 26: Java Web Applications - Servlet Application Architecture - Servlet API Overview - Servlet - Writing a Basic Servlet Application - ServletRequest - ServletResponse - ServletConfig - ServletContext - GenericServlet - HTTP Servlets - Using the Deployment Descriptor - Summary - Questions Chapter 27: JavaServer Pages - A JSP Overview - jspInit, jspDestroy, and Other Methods - Implicit Objects - JSP Syntactic Elements - Handling Errors - Summary - Questions Chapter 28: Javadoc - Writing Documentation in Java Classes - Javadoc Syntax - Summary - Question Chapter 29: Application Deployment - JWS Overview - JNLP File Syntax - A Deployment Example - Security Concerns - Summary - Questions Chapter 30: Reflection - Overview - java.lang.Class - Creating An Object - Creating An Array - Field Manipulation - Method Manipulation - Invoking A Method - Summary - Questions Chapter 31: Introduction to JavaFX - Overview - Setting up - Your First JavaFX Application - Application, Stage, and Scene - UI Components - Controls - Regions - Event Handling - Styling with CSS - Summary - Questions Chapter 32: JavaFX with FXML - Overview - A Simple FXML-Based Application - Event Handling with FXML - Summary - Questions Chapter 33: Introduction to Android Programming - Overview - Downloading and Installing Android Development Tools - Your First Android Application - The Android Manifest - Running An Application on the Emulator - Application Structure - Changing the Application Icon - Logging - Debugging An Application - Running on A Real Device - Upgrading the SDK - Summary - Questions Chapter 34: Creating Android Applications - Overview - The Activity Lifecycle - Android UI Components - Layouts - Listeners - Starting Another Activity - Summary - Questions Chapter 35: More Android Applications - Handling the Handler - MediaRecorder - Asynchronous Tasks - Summary - Questions Appendix A: javac - Options - Command Line Argument Files Appendix B: java - Options Appendix C: jar - Syntax - Options - Examples - Setting an Application's Entry Point Appendix D: NetBeans - Download and Installation - Creating a Project - Creating a Class - Running a Java Class - Adding Libraries - Debugging Code Appendix E: Eclipse - Download and Installation - Creating a Project - Creating a Class - Running a Java Class - Adding Libraries - Debugging Code