Visual Basic: A Beginner's Tutorial
by Jayden Ky
ISBN: 9780992133023
$39.99, 280 pages
by Jayden Ky
ISBN: 9780992133023
$39.99, 280 pages
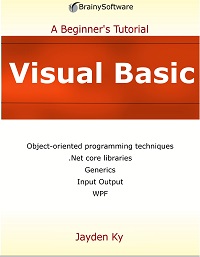
Designed as a beginner's tutorial to the latest version of Visual Basic, this informative guide discusses the most important features of the language and teaches how to use the .NET Framework. Written with clarity and readability in mind, it introduces important programming concepts and explains the process of building real-world applications, both desktop and web-based. With the most comprehensive coverage possible in a book for beginners, it includes such topics as VB language syntax, object-oriented programming, working with numbers and dates, error handling, input output, generics, annotations, database access, security, and application deployment.
Table of Contents
Introduction - Overview of the .NET Framework - An Overview of Object-Oriented Programming - About This Book - Downloading and Installing the .NET Framework - Choosing An IDE - Downloading Program Examples Chapter 1: Your First Taste of Visual Basic - Your First Visual Basic Program - Visual Basic Code Conventions - Summary Chapter 2: Language Fundamentals - ASCII and Unicode - Intrinsic Types and the Common Type System - Variables - Constants - Literals - Primitive Conversions - Operators - Comments - Summary Chapter 3: Statements - An Overview of Visual Basic Statements - The if Statement - The while Statement - The do-while Statement - The for Statement - The break Statement - The continue Statement - The switch Statement - Summary Chapter 4: Objects and Classes - What Is an Object? - VB Classes - Creating Objects - The null Keyword - Objects in Memory - Namespaces - Encapsulation and Class Access Control - The this Keyword - Using Other Classes - Static Members - Variable Scope - Method Overloading - Summary Chapter 5: Core Classes - System.Object - System.String - System.Text.StringBuilder - Arrays - System.Console - Summary Chapter 6: Inheritance - An Overview of Inheritance - Accessibility - Method Overriding - Calling the Base Class?s Constructors - Calling the Base Class?s Hidden Members - Type Casting - Sealed Classes - The is Keyword - Summary Chapter 7: Structures - An Overview of Structures - .NET Structures - Writing A Structure - Nullable Types - Summary Chapter 8: Error Handling - Catching Exceptions - try without catch and the using Statement - The System.Exception Class - Throwing an Exception from a Method - Final Note on Exception Handling - Summary Chapter 9: Numbers and Dates - Number Parsing - Number Formatting - The System.Math Class - Working with Dates and Times - Summary Chapter 10: Interfaces and Abstract Classes - The Concept of Interface - The Interface, Technically Speaking - Implementing System.IComparable - Abstract Classes - Summary Chapter 11: Enumerations - An Overview of Enum - Enums in a Class - Switching on enum - Summary Chapter 12: Generics - Why Generics? - Introducing Generic Types - Applying Restrictions - Writing Generic Types - Summary Chapter 13: Collections - Overview - The List Class - The HashSet Class - The Queue Class - The Dictionary Class - Summary Chapter 14: Input/Output - File and Directory Handling and Manipulation - Input/Output Streams - Reading Text (Characters) - Writing Text (Characters) - Reading and Writing Binary Data - Summary Chapter 15: Windows Presentation Foundation - Overview - Application and Window - WPF Controls - Panels and Layout - Event Handling - XAML - Summary Chapter 16: Polymorphism - Defining Polymorphism - Polymorphism in Action - Polymorphism in a Drawing Application - Summary Chapter 17: ADO.NET - Introduction to ADO.NET - Five Steps to Data Access - Connecting to SQL Server Example - Summary Appendix A: Visual Studio Express 2013 for Windows Desktop - Hardware and Software Requirements - Download and Installation - Registering Visual Studio Express 2012 - Creating a Project - Creating a Class - Running a Project Appendix B: SQL Server 2012 Express